Let's learn how to create a featured video feature for WordPress without a plugin.
You must edit your WordPress Theme code and add some extra fields on the page or post options.
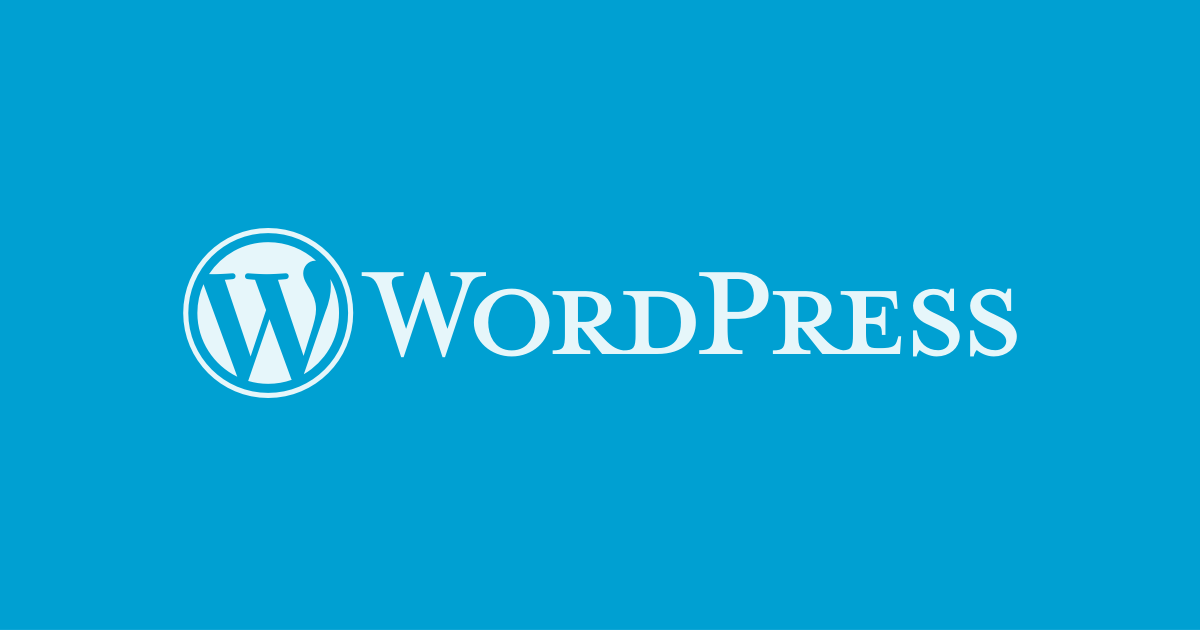
Add Featured Video Field on the Post and Pages.
First, you must write the code below into the functions.php file of the theme.
<?php // Add the Page Meta Box function codeless_add_custom_meta_box() { add_meta_box( 'codeless_meta_box', // $id 'Codeless Page Options', // $title 'codeless_show_custom_meta_box', // $callback 'page', // $page 'normal', // $context 'high'); // $priority } add_action('add_meta_boxes', 'codeless_add_custom_meta_box'); // Add the Post Meta Box function codeless_add_custom_post_meta_box() { add_meta_box( 'codeless_meta_box', // $id 'Codeless Page Options', // $title 'codeless_show_custom_post_meta_box', // $callback 'post', // $post 'normal', // $context 'high'); // $priority } add_action('add_meta_boxes', 'codeless_add_custom_post_meta_box'); $prefix = 'codeless_'; // Field Array (Pages Meta) $codeless_meta_fields = array(); // Field Array (Posts Meta) $codeless_post_meta_fields = array( array( 'label' => 'Featured Video Embed Code', 'desc' => 'Paste your video code here to show a video instead of a featured image.', 'id' => $prefix . 'video_embed', 'type' => 'textarea' ) ); // The Callback for page meta box function codeless_show_custom_meta_box() { global $codeless_meta_fields; codeless_show_page_meta_box($codeless_meta_fields); } // The Callback for post meta box function codeless_show_custom_post_meta_box() { global $codeless_post_meta_fields; codeless_show_page_meta_box($codeless_post_meta_fields); } // The Callback function codeless_show_page_meta_box($meta_fields) { global $post; // Use nonce for verification echo '<input type="hidden" name="custom_meta_box_nonce" value="' . wp_create_nonce(basename(__FILE__)) . '" />'; // Begin the field table and loop echo '<table class="form-table">'; foreach ($meta_fields as $field) { // get value of this field if it exists for this post $meta = get_post_meta($post->ID, $field['id'], true); // begin a table row with echo '<tr> <th><label for="' . $field['id'] . '">' . $field['label'] . '</label></th> <td>'; switch ($field['type']) { // text case 'text': echo '<input type="text" name="' . $field['id'] . '" id="' . $field['id'] . '" value="' . $meta . '" style="width:100%" /> <br /><span class="description">' . $field['desc'] . '</span>'; break; // textarea case 'textarea': echo '<textarea style="width:100%" rows="2" id="' . $field['id'] . '" name="' . $field['id'] . '">' . $meta . '</textarea> <br /><span class="description">' . $field['desc'] . '</span>'; break; // checkbox case 'checkbox': echo '<input type="checkbox" name="' . $field['id'] . '" id="' . $field['id'] . '" ', $meta ? ' checked="checked"' : '', '/> <label for="' . $field['id'] . '">' . $field['desc'] . '</label>'; break; // select case 'select': echo '<select name="' . $field['id'] . '" id="' . $field['id'] . '">'; foreach ($field['options'] as $option) { echo '<option', $meta == $option['value'] ? ' selected="selected"' : '', ' value="' . $option['value'] . '">' . $option['label'] . '</option>'; } echo '</select><br /><span class="description">' . $field['desc'] . '</span>'; break; } //end switch echo '</td></tr>'; } // end foreach echo '</table>'; // end table } // Save the Data function codeless_save_custom_meta($post_id) { global $codeless_meta_fields; global $codeless_post_meta_fields; // verify nonce if (!wp_verify_nonce($_POST['custom_meta_box_nonce'], basename(__FILE__))) return $post_id; // check autosave if (defined('DOING_AUTOSAVE') && DOING_AUTOSAVE) return $post_id; // check permissions if ('page' == $_POST['post_type']) { if (!current_user_can('edit_page', $post_id)) return $post_id; } elseif (!current_user_can('edit_post', $post_id)) { return $post_id; } //either post or page fields we'll be working with $fields; // Check permissions (pages or posts) if ('page' == $_POST['post_type']) { $fields = $codeless_meta_fields; } else if ('post' == $_POST['post_type']) { $fields = $codeless_post_meta_fields; } // loop through fields and save the data foreach ($fields as $field) { $old = get_post_meta($post_id, $field['id'], true); $new = $_POST[$field['id']]; if ($new && $new != $old) { update_post_meta($post_id, $field['id'], $new); } elseif ('' == $new && $old) { delete_post_meta($post_id, $field['id'], $old); } } // end foreach } add_action('save_post', 'codeless_save_custom_meta'); ?>
Editing the theme template file
You have to find the function that is responsible for the featured image: the_post_thumbnail()
In the case of Codeless Themes, the file you have to edit is: wp-content/themes/folie/template-parts/blog/parts/style-default.php file
if ( has_post_thumbnail() && $post_format != 'gallery' && ( ! is_single() || is_single() ) ) : get_template_part( 'template-parts/blog/parts/entry', 'thumbnail' ); endif; ?>
replace with:
if ( has_post_thumbnail() && $post_format != 'gallery' && ( ! is_single() || is_single() ) ) get_template_part( 'template-parts/blog/parts/entry', 'thumbnail' ); else if (get_post_meta(get_the_ID(), 'codeless_video_embed', true)) { ?> <!-- show the featured video--> <div class="videoWrapper"> <?php echo get_post_meta(get_the_ID(), 'codeless_video_embed', true); ?> </div> <?php } else { ?> <!--if no featured image or thumbnail, do something...--> <?php } ?>
Save the file.
Now in the post or pages, you will find a new field to add the featured video.
In case you don't want to write the above you can also use plugins like:

Ludjon, who co-founded Codeless, possesses a deep passion for technology and the web. With over a decade of experience in constructing websites and developing widely-used WordPress themes, Ludjon has established himself as an accomplished expert in the field.
Comments
You’re a really useful site; could not make it without ya!
I’m really pleased to say it was an interesting post to read.
King regards,
Demir Zacho
Enjoyed examining this, very good stuff, appreciate it.
Great looking site. Assume you did a whole lot of your very own coding.
If the theme is updated is this going to change?
Hello,
You can add the code in the child-theme template to not lose the changes after the update.
Regards!
hi bro , please make code snipp for auto set first video tag src find in content as fetured image & auto play as scroll on it.
waiting for your repsonse
finest regards