Learning to deploy a Next.js application on cPanel in a manner that's seamless and effective. I'll guide you step-by-step in this process.
Before diving in, let's unpack what Next.js and cPanel are, just in case you're new to these terms.
What is Next.js
Next.js is a neat framework, based on React.js. It's a wizard when it comes to building server-side rendering (SSR) apps. React.js only supports making single-page applications (SPA) by default. However, Next.js leaps in with its attractive SSR feature. What else? Next.js packs a punch with a file-based routing system, among other perks.
What is cPanel
Moving to cPanel. Think of it as software simplifying server management. It brings to your table a host of tools. File Manager, Database Manager, and Domain Name Manager are just a few examples.
Now that we've made friends with Next.js and cPanel, let's crack on with our main mission: deploying a Next.js app on cPanel. Assuming you've got a Next.js app, a web host with cPanel, and a linked domain name, let's march ahead.
Here I have listed the top best Next.Js hosting providers.
Deploy Next.js app to cPanel
Step 1: Begin by setting up a custom Next.js server. You need a server.js file in your project's root directory, populated with the needed code. Look up an official Next.js guide for help.
const { createServer } = require('http')
const { parse } = require('url')
const next = require('next')
const dev = process.env.NODE_ENV !== 'production'
const hostname = 'localhost'
const port = 3000
// when using middleware `hostname` and `port` must be provided below
const app = next({ dev, hostname, port })
const handle = app.getRequestHandler()
app.prepare().then(() => {
createServer(async (req, res) => {
try {
// Be sure to pass `true` as the second argument to `url.parse`.
// This tells it to parse the query portion of the URL.
const parsedUrl = parse(req.url, true)
const { pathname, query } = parsedUrl
if (pathname === '/a') {
await app.render(req, res, '/a', query)
} else if (pathname === '/b') {
await app.render(req, res, '/b', query)
} else {
await handle(req, res, parsedUrl)
}
} catch (err) {
console.error('Error occurred handling', req.url, err)
res.statusCode = 500
res.end('internal server error')
}
})
.once('error', (err) => {
console.error(err)
process.exit(1)
})
.listen(port, () => {
console.log(`> Ready on http://${hostname}:${port}`)
})
})
Step 2: Tweak the package.json file. This step makes the environment ‘production-ready' and activates the server.js file.
{
"scripts": {
"start": "NODE_ENV=production node server.js"
}
}
Step 3: Time to build your Next.js application. You can do this with the npm run build or yarn run build command in your Terminal.
Step 4: Post-build, locate your Next.js project files in your file manager. If you don't see hidden files, turn on the visibility. Avoid node_modules and .git folders, README.md, and .gitignore files. Select all other files and folders, and create a ZIP file.
Step 5: Now, log into your cPanel web hosting. Upload and extract the ZIP file to your domain name's root folder.
Step 6: Your project files are ready. Head over to the Software section in your cPanel. Click on Setup Node.js App, followed by + Create Application button. Set up your Node.js app, keeping in mind the Node.js version, production application mode, application root, URL, and startup file (server.js).
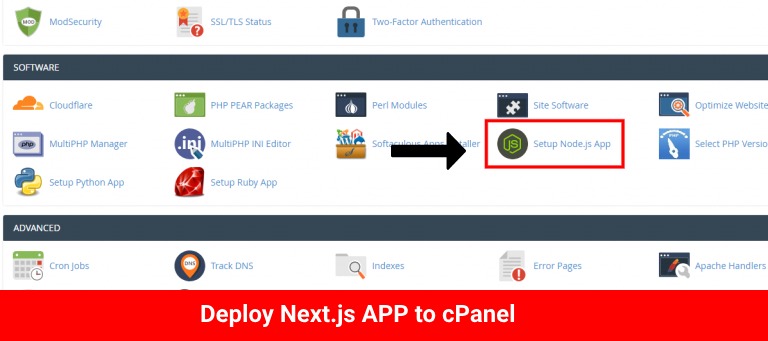
Finish by clicking CREATE. Once created, stop it for a while. Scroll down to the Detected configuration files section. Run NPM Install to get all Node.js packages. Finally, click START APP.
There you go! Open your domain name in your browser. Your Next.js app is now live. Great job on the successful deployment!
Sharing this method of deploying Next.js apps on cPanel was a joy. Best of luck and happy coding!
Pros and Cons of Deploying a NextJs to cPanel
Deploying a Next.js application to cPanel comes with a variety of benefits. Here are some pros and cons to consider.
Pros:
- User-Friendly: cPanel provides an easy-to-use graphical interface, which simplifies the deployment process.
- Automation: cPanel includes many automated tools that can handle tasks such as creating databases, managing website files, setting up email, and more.
- Versatility: cPanel supports a wide range of applications, including Node.js apps such as those built with Next.js.
Cons:
- Limited Flexibility: Although cPanel is easy to use, it can sometimes limit flexibility and control over your server environment due to its simplified interface.
- Potential Compatibility Issues: Some users have reported problems when deploying Next.js applications on shared hosting platforms, such as running into errors during the build process.
FAQs
Hosting a Next.js website on cPanel can have several advantages, such as cost savings and the retention of Next.js capabilities. However, there are some limitations to be aware of. For example, cPanel does not support serverless functions and automatic static optimization. The decision to host on cPanel should be based on individual preferences and business needs.
The .htaccess file configuration is a critical part of deploying a Next.js application on cPanel. This file controls the server's behavior when it encounters certain conditions. The specific configuration provided in the example helps to handle requests and redirections properly.
If you experience crashes or redirection issues when deploying your Next.js app on cPanel, one solution could be creating a startup file named app.js in your root folder. You need to run your application as a Node.js application in this scenario.
Disabling image optimization when deploying a Next.js app on cPanel can be done, but the exact instructions were not included in the provided information. For specific steps, you should refer to the official Next.js documentation or relevant tutorials.
Other Resources
Best Next.JS Hosting Providers
Best Node.js Hosting Providers
Conclusion
While deploying a Next.js application to cPanel may come with a few challenges, the benefits often outweigh the drawbacks. The simplicity and automation offered by cPanel make the deployment process straightforward and manageable, even for developers who are new to the process. With this guide, you should now be better equipped to deploy your Next.js applications to cPanel.

As one of the co-founders of Codeless, I bring to the table expertise in developing WordPress and web applications, as well as a track record of effectively managing hosting and servers. My passion for acquiring knowledge and my enthusiasm for constructing and testing novel technologies drive me to constantly innovate and improve.
Expertise:
Web Development,
Web Design,
Linux System Administration,
SEO
Experience:
15 years of experience in Web Development by developing and designing some of the most popular WordPress Themes like Specular, Tower, and Folie.
Education:
I have a degree in Engineering Physics and MSC in Material Science and Opto Electronics.
Comments
Hello what version of next are you using?
I am using version 13 and with cpanel I have had many problems
Try to use latest version of Node.JS because cPanel offers it. Maybe this udpate can resolve your issues.
will this steps change if iam using next js 13 app directory