The HTTP 499 status code, known as “Client Closed Request,” is an error that occurs when a client—typically a web browser or API client—closes the connection before the server finishes processing the request. This status code is unique to NGINX and does not belong to the official HTTP status codes, but it's essential to understand and address it when encountered.
In this article, we'll explore what the 499 status code means, its common causes, how to diagnose it, and steps to prevent and fix the issue.
What is HTTP 499 Status Code?
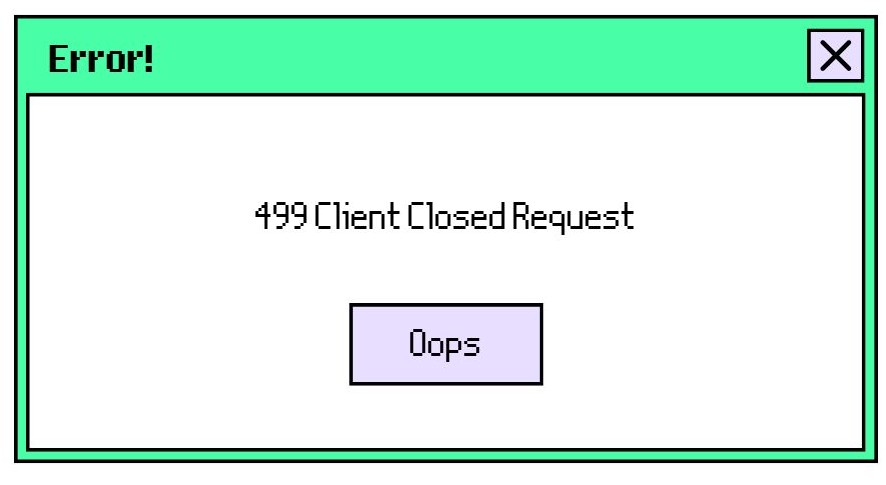
The HTTP 499 status code is specific to NGINX web servers. It indicates that the client decided to terminate the connection before the server could deliver the requested response. This often leads to incomplete data transmission, causing errors in websites, APIs, or other server-based systems.
Common Causes of 499 Status Code
There are several reasons why a 499 Client Closed Request error might occur. Here are the most common ones:
- Slow server response time: If the server takes too long to process a request, the client may lose patience and close the connection.
- Client timeout settings: Some clients are configured to terminate connections after a specific time limit.
- Network interruptions: Disruptions in network connectivity, such as Wi-Fi drops or server overloads, may cause the client to close the connection.
- Large data transfers: Requests involving significant data exchanges, like large file uploads or API queries, may take longer to process, leading the client to close the request prematurely.
How to Diagnose HTTP 499 Status Code Errors
Diagnosing the 499 status code error requires some analysis of server logs and network behavior. Here are some steps to help identify the cause:
- Check server logs: Start by inspecting the NGINX logs. You'll find information about requests that were prematurely closed by the client, including IP addresses, request times, and specific URLs involved.
- Monitor server performance: Check the server’s performance metrics. High load, slow response times, or excessive requests could indicate an overwhelmed server, which may cause clients to disconnect.
- Review client-side behavior: Identify whether the issue is related to specific clients or locations. A pattern in the source of the requests can provide clues about network connectivity or client-side timeouts.
- Use network tools: Tools like Wireshark or network analyzers can help track packet loss or latency, identifying any possible network interruptions causing the issue.
How to Prevent HTTP 499 Errors
Prevention is key to reducing the occurrence of 499 errors. Here are a few strategies to minimize the chances of encountering this issue:
- Optimize server response times: Speed up the server’s processing time by optimizing code, queries, or database calls. A faster server means a lower likelihood of clients terminating requests due to long delays.
- Set reasonable timeouts: Adjust the client-side and server-side timeout settings. Increasing the timeout limit can give the server more time to process the request before the client closes the connection.
- Improve network reliability: Ensure that the network infrastructure is stable and reliable. Frequent network issues may frustrate clients, leading them to disconnect prematurely.
- Use content delivery networks (CDNs): Implementing CDNs can distribute the load and deliver content faster to users, decreasing the likelihood of 499 errors.
How to Fix HTTP 499 Status Code from the Browser Side
This title clearly conveys that the focus is on fixing the 499 status code specifically from the browser's perspective, offering actionable solutions for users and developers.
1. Increase Browser Timeout for Long-Running Requests
Browsers can time out and close connections if the server takes too long to respond. While modern browsers typically handle timeouts internally, certain network configurations or JavaScript requests might have their own timeout settings that can be adjusted.
Fix for Users: Adjust Browser Timeout Settings
Some browsers allow advanced configuration for network timeouts:
- Google Chrome: You can't directly modify the HTTP timeout, but you can reduce network load by closing unused tabs, clearing cache, or using browser extensions that manage timeouts.
- Mozilla Firefox: You can adjust the network timeout settings via
about:config
:- Open Firefox and type
about:config
in the address bar. - Search for the key
network.http.connection-timeout
. - Increase the value (default is 90 seconds). Set it to something higher, like
300
, to give the server more time.
- Open Firefox and type
Fix for Developers: Handle Client-Side Timeouts in JavaScript
For JavaScript-based requests, you can modify client-side timeout behavior in requests that involve fetching data from servers. For instance, in AJAX requests (using XMLHttpRequest
) or fetch()
, you can specify longer timeouts.
2. Use Keep-Alive to Maintain Connection
Modern browsers support Keep-Alive headers, which help keep the connection between the client and server open, reducing the risk of prematurely closing the connection. Using Keep-Alive allows the client and server to reuse the same connection, which can be helpful for long-running processes or multiple small requests.
Fix for Developers: Implement Keep-Alive in HTTP Requests
Ensure that the browser is using Keep-Alive in its headers for connections:
fetch('https://example.com/api/data', {
headers: {
'Connection': 'keep-alive'
}
});
This header can prevent the browser from closing the connection too soon, especially in cases where the server takes longer to respond.
3. Ensure Stable Network Connectivity
Intermittent or unstable network connections can cause the browser to drop requests, leading to 499 errors. Checking and optimizing network conditions is crucial for reducing these issues.
Fix for Users: Ensure Strong Internet Connection
- Make sure you're connected to a stable and fast internet connection. Switching from Wi-Fi to a wired Ethernet connection may improve stability.
- Avoid heavy background network activities that can disrupt connectivity (e.g., downloading large files while browsing).
Fix for Developers: Use Network Monitoring Tools
For users experiencing 499 errors due to network drops, developers can monitor network conditions using Network Information API and alert users when the connection is unstable.
javascriptCopy codeif (navigator.connection) {
const connection = navigator.connection.effectiveType;
if (connection === '2g' || connection === 'slow-2g') {
alert('Your network connection is slow, which may cause requests to fail.');
}
}
This notifies users when their network might cause issues, preventing them from closing connections prematurely.
4. Clear Browser Cache and Cookies
Sometimes, a corrupted cache or stale cookies can cause connection errors, including 499 status codes. Clearing the browser cache and cookies might help resolve persistent client-side disconnections.
Fix for Users: Clear Cache and Cookies
To clear cache and cookies in your browser:
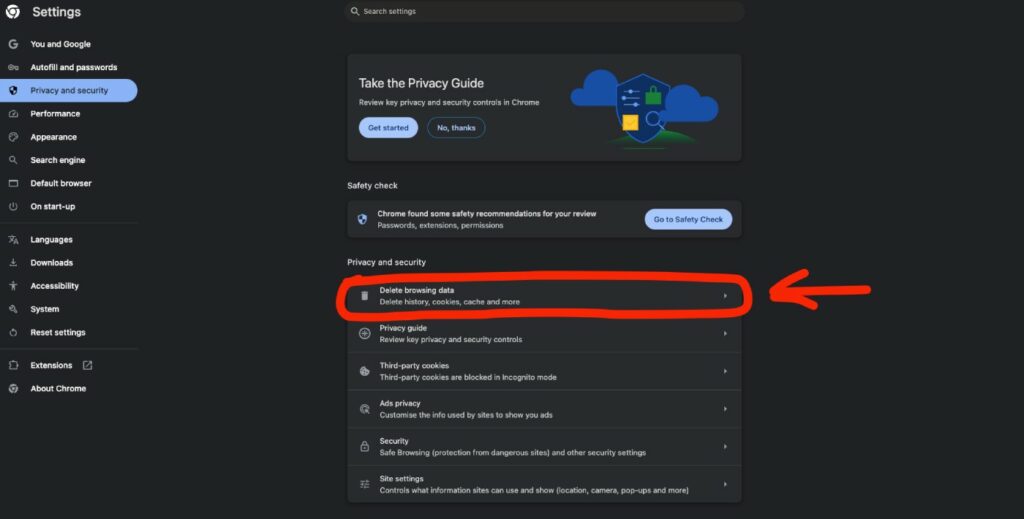
- Google Chrome:
- Open Chrome and go to the menu (
⋮
). - Navigate to Settings> Privacy and Security > Delete Browsing Data
- Select Cached images and files and Cookies and other site data.
- Click Delete data.
- Open Chrome and go to the menu (
- Mozilla Firefox:
- Go to the menu (
≡
) and select Settings. - Under Privacy & Security, scroll to Cookies and Site Data and click Clear Data.
- Go to the menu (
Clearing the cache ensures that no outdated or corrupt data interferes with the connection process.
5. Reduce Tab and Browser Extensions Overload
Running multiple tabs or having too many browser extensions active can strain the browser's resources and lead to premature request closures.
Fix for Users: Close Unused Tabs and Disable Unnecessary Extensions
- Close Unused Tabs: Close any unnecessary tabs to free up browser memory and network resources. This can prevent the browser from forcefully closing requests due to resource limitations.
- Disable Unused Extensions:
- In Chrome: Go to the Extensions menu (
⋮
> Extensions > Manage Extensions ), and disable extensions that aren’t required. - In Firefox: Go to Add-ons Manager (
≡
> Add-ons > Extensions), and disable any non-essential extensions.
- In Chrome: Go to the Extensions menu (
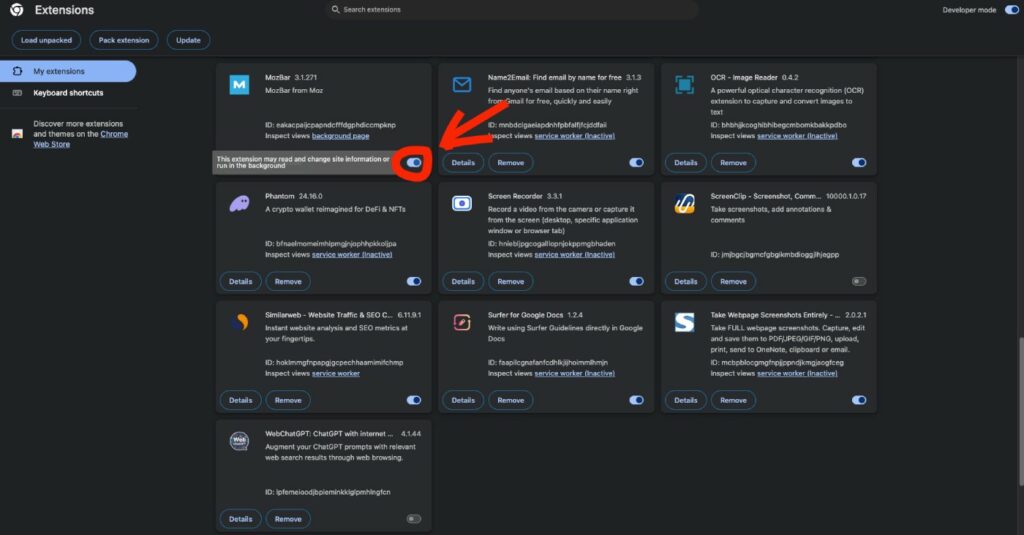
Disabling resource-heavy extensions (like ad blockers or certain developer tools) can reduce the load on the browser and help prevent 499 errors.
6. Monitor Client-Side Errors Using Developer Tools
Most modern browsers come with built-in developer tools that can help you monitor network activity and detect 499 status codes or other connection-related issues.
Fix for Users: Use Network Tab in Developer Tools
To troubleshoot client-side issues that might cause 499 errors, use the browser’s Developer Tools to check for network issues:
- Google Chrome:
- Press
Ctrl + Shift + I
or right-click on the page and select Inspect. - Go to the Network tab to monitor active requests.
- Look for failed requests with 499 and check the Time column to see if a long request duration caused the issue.
- Press
- Mozilla Firefox:
- Press
Ctrl + Shift + I
to open Developer Tools. - Navigate to the Network tab and filter by 499 to monitor client-terminated requests.
- Press
Using this information, users or developers can identify if slow server responses or client-side errors are causing the problem.
How to Fix 499 Status Code Error on Server Sider
If you're encountering 499 Client Closed Request errors frequently, here's how to fix them if you are the administrator:
1. Increase Client Timeout Settings
In many cases, the client closes the connection because the server takes too long to respond. Increasing the timeout setting on the client side can help prevent this.
Example: Adjusting Timeout in NGINX
If you're using an API and the client is closing the request due to a long response time, adjust the timeout settings on the server side. For NGINX, you can modify the following settings in the NGINX configuration file (nginx.conf
):
http {
...
client_header_timeout 300s;
client_body_timeout 300s;
send_timeout 300s;
...
}
These values set the timeout for reading client requests and sending responses to 300 seconds, allowing more time for slower clients or larger data transfers.
2. Optimize Server-Side Performance
Sometimes, the server takes too long to process a request, causing the client to terminate the connection. Optimizing server-side code and databases can speed up response times and reduce 499 errors.
Example: Optimize Database Queries
If your server is spending too much time on database queries, consider optimizing SQL queries. For example, instead of making multiple queries to retrieve data from different tables, you could use JOINs to reduce the time taken for database operations:
SELECT orders.order_id, customers.customer_name
FROM orders
JOIN customers ON orders.customer_id = customers.customer_id
WHERE orders.order_date > '2023-01-01';
This single query combines data from both the orders
and customers
tables, minimizing the number of database calls.
3. Implement Retry Logic in Clients
If your client is closing requests due to network hiccups or long server processing times, you can implement retry logic to handle transient issues without requiring manual intervention.
Example: Retry Logic in an API Client (Python)
Here’s how you can implement retry logic using the requests
library in Python:
import requests
from requests.adapters import HTTPAdapter
from urllib3.util.retry import Retry
# Set up retry logic
retry_strategy = Retry(
total=3, # Retry a total of 3 times
status_forcelist=[499], # Retry on 499 status code
method_whitelist=["GET", "POST"], # Retry for specific methods
backoff_factor=1 # Wait time between retries (exponential backoff)
)
adapter = HTTPAdapter(max_retries=retry_strategy)
http = requests.Session()
http.mount("https://", adapter)
try:
response = http.get("https://example.com/api/data")
response.raise_for_status() # Raise error if status code is not 2xx
except requests.exceptions.RequestException as e:
print(f"Request failed: {e}")
This retry logic automatically resends requests up to 3 times if a 499 error occurs.
4. Use Load Balancing and CDNs
Overloaded servers often cause clients to terminate requests prematurely. Distributing the load using a load balancer or Content Delivery Network (CDN) can help mitigate the issue.
Example: Configuring Load Balancing in NGINX
If you're using NGINX as a load balancer, you can distribute the load across multiple servers to avoid slow responses:
http {
upstream backend_servers {
server backend1.example.com;
server backend2.example.com;
}
server {
location / {
proxy_pass http://backend_servers;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
}
This configuration directs requests to two backend servers (backend1.example.com
and backend2.example.com
), balancing the load and reducing response time.
5. Improve Network Stability
Intermittent network issues can cause clients to lose connection with the server, leading to 499 errors. Ensuring reliable network infrastructure is critical.
Example: Monitor Network Health
Use tools like Pingdom, Nagios, or Wireshark to monitor network stability. If network latency or packet loss is high, contact your network administrator or Internet Service Provider (ISP) to resolve the underlying issues.
6. Cache Content to Speed Up Responses
If your server delivers the same content to multiple clients, you can use caching to speed up the process and reduce the load on the server.
Example: Enable Caching in NGINX
To enable caching in NGINX, you can configure it to cache responses for repeated requests:
http {
proxy_cache_path /data/nginx/cache levels=1:2 keys_zone=my_cache:10m max_size=10g inactive=60m use_temp_path=off;
server {
location / {
proxy_cache my_cache;
proxy_pass http://backend_servers;
add_header X-Cache-Status $upstream_cache_status;
}
}
}
This configuration caches responses from backend servers, allowing subsequent requests to be served quickly from the cache, reducing the load on your backend.
7. Avoid Large Payloads or Optimize Data Transmission
If large payloads are causing the client to close the connection prematurely, consider compressing or chunking the data for transmission.
Example: Enable Gzip Compression
You can enable Gzip compression in NGINX to reduce the size of responses, speeding up transmission and reducing the chance of 499 errors:
http {
gzip on;
gzip_types text/plain text/css application/json application/javascript;
gzip_min_length 1000;
}
This compresses the response if it’s larger than 1000 bytes, which can make the data transfer faster and prevent client timeouts.
Similar errors
522 Error: How to Troubleshoot and Fix
HTTP 403 “Forbidden”: Causes, Prevent and Fix
Conclusion
The 499 status code, while not part of the official HTTP protocol, is crucial for diagnosing client-server communication issues. By understanding the causes, diagnosing effectively, and implementing the proper prevention and fixes, you can significantly reduce the occurrence of these errors and improve the user experience.

As one of the co-founders of Codeless, I bring to the table expertise in developing WordPress and web applications, as well as a track record of effectively managing hosting and servers. My passion for acquiring knowledge and my enthusiasm for constructing and testing novel technologies drive me to constantly innovate and improve.
Expertise:
Web Development,
Web Design,
Linux System Administration,
SEO
Experience:
15 years of experience in Web Development by developing and designing some of the most popular WordPress Themes like Specular, Tower, and Folie.
Education:
I have a degree in Engineering Physics and MSC in Material Science and Opto Electronics.
Comments